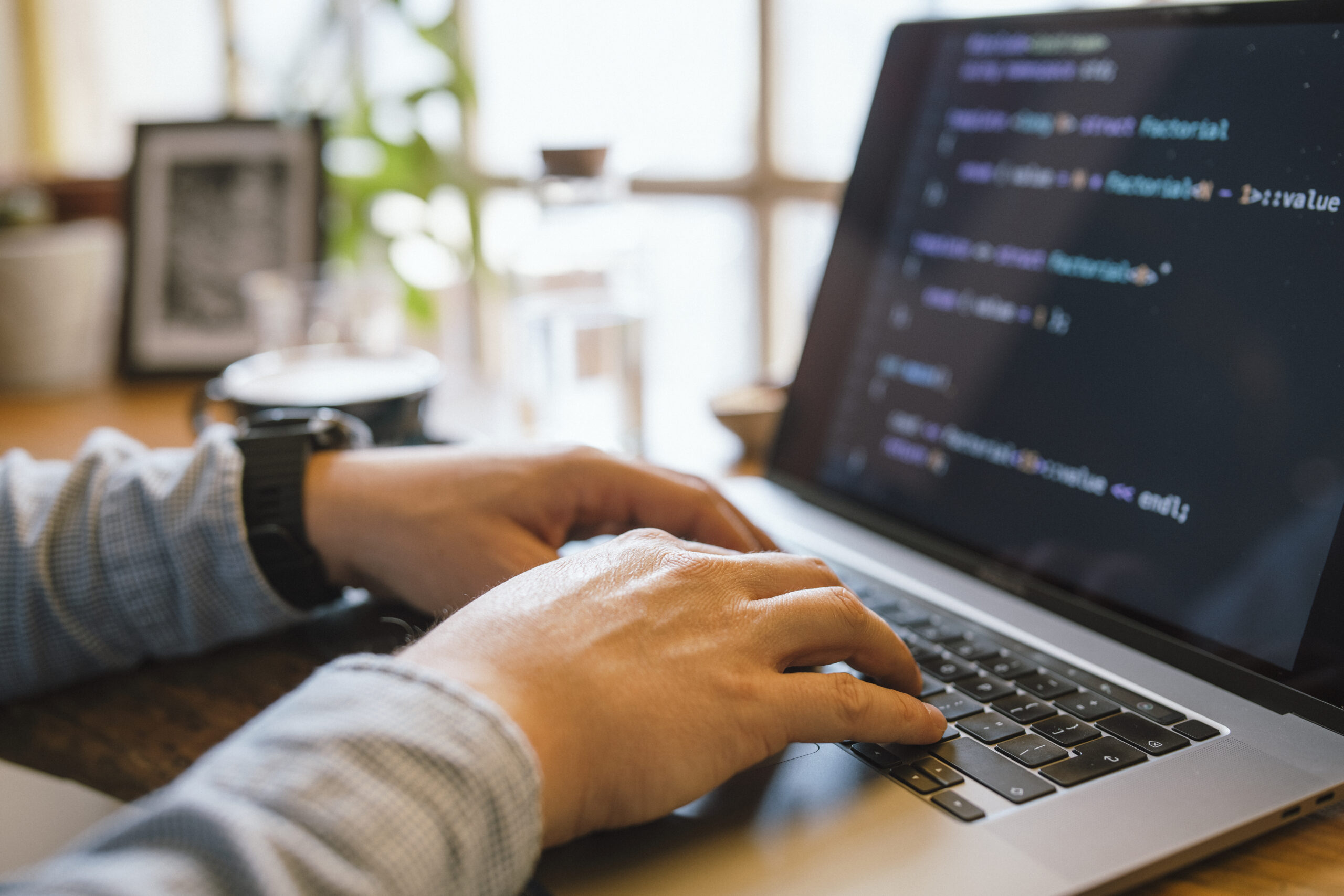
Debugging is Just about the most crucial — nevertheless normally ignored — capabilities in a very developer’s toolkit. It isn't really pretty much repairing damaged code; it’s about knowledge how and why matters go wrong, and Studying to Feel methodically to unravel complications competently. Whether you're a beginner or a seasoned developer, sharpening your debugging techniques can help save hours of annoyance and radically improve your productiveness. Here's various strategies to help builders amount up their debugging sport by me, Gustavo Woltmann.
Master Your Tools
Among the quickest methods developers can elevate their debugging expertise is by mastering the applications they use everyday. Though crafting code is a person Component of growth, recognizing ways to communicate with it efficiently in the course of execution is equally vital. Modern enhancement environments appear equipped with powerful debugging abilities — but several builders only scratch the floor of what these resources can perform.
Just take, as an example, an Built-in Growth Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the worth of variables at runtime, step via code line by line, and perhaps modify code about the fly. When utilized effectively, they Allow you to notice just how your code behaves throughout execution, and that is invaluable for monitoring down elusive bugs.
Browser developer tools, for example Chrome DevTools, are indispensable for entrance-conclusion developers. They help you inspect the DOM, keep track of community requests, view true-time functionality metrics, and debug JavaScript during the browser. Mastering the console, sources, and community tabs can transform aggravating UI difficulties into manageable responsibilities.
For backend or method-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate above functioning processes and memory management. Finding out these applications may have a steeper Understanding curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, turn out to be cozy with Model Command systems like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic modifications.
Eventually, mastering your instruments suggests likely further than default settings and shortcuts — it’s about creating an personal familiarity with your progress ecosystem so that when problems arise, you’re not misplaced in the dead of night. The higher you already know your instruments, the greater time you may shell out fixing the particular trouble rather then fumbling as a result of the procedure.
Reproduce the situation
Among the most important — and infrequently missed — ways in productive debugging is reproducing the situation. In advance of jumping in to the code or generating guesses, developers require to produce a dependable natural environment or situation exactly where the bug reliably appears. With out reproducibility, correcting a bug will become a match of likelihood, often bringing about squandered time and fragile code alterations.
The first step in reproducing a dilemma is collecting as much context as feasible. Question issues like: What actions led to The problem? Which atmosphere was it in — development, staging, or generation? Are there any logs, screenshots, or error messages? The more depth you've, the a lot easier it gets to isolate the exact ailments below which the bug occurs.
When you finally’ve collected more than enough details, try to recreate the challenge in your local ecosystem. This might necessarily mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty seems intermittently, consider composing automatic tests that replicate the sting cases or state transitions involved. These exams don't just assist expose the situation but also avoid regressions Down the road.
From time to time, the issue could possibly be ecosystem-certain — it would materialize only on particular working methods, browsers, or beneath unique configurations. Using resources like Digital devices, containerization (e.g., Docker), or cross-browser tests platforms could be instrumental in replicating these bugs.
Reproducing the condition isn’t just a stage — it’s a attitude. It involves tolerance, observation, and a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to fixing it. Having a reproducible circumstance, You may use your debugging applications more successfully, check prospective fixes securely, and talk much more Obviously using your crew or end users. It turns an abstract complaint into a concrete challenge — and that’s exactly where developers prosper.
Browse and Have an understanding of the Mistake Messages
Mistake messages tend to be the most respected clues a developer has when one thing goes Improper. As opposed to seeing them as frustrating interruptions, builders really should discover to take care of mistake messages as direct communications in the method. They often show you just what exactly transpired, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by studying the information carefully As well as in total. Many builders, particularly when under time force, glance at the initial line and immediately start out producing assumptions. But deeper from the error stack or logs may perhaps lie the real root lead to. Don’t just copy and paste mistake messages into engines like google — study and fully grasp them very first.
Crack the error down into components. Can it be a syntax error, a runtime exception, or maybe a logic error? Will it point to a certain file and line number? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re applying. Error messages in languages like Python, JavaScript, or Java generally adhere to predictable designs, and Discovering to recognize these can substantially speed up your debugging system.
Some mistakes are obscure or generic, As well as in those situations, it’s very important to examine the context during which the error occurred. Check out similar log entries, input values, and recent alterations during the codebase.
Don’t neglect compiler or linter warnings both. These often precede greater troubles and supply hints about opportunity bugs.
In the end, error messages will not be your enemies—they’re your guides. Mastering to interpret them appropriately turns chaos into clarity, supporting you pinpoint difficulties faster, reduce debugging time, and become a much more productive and self-confident developer.
Use Logging Correctly
Logging is Probably the most effective equipment in the developer’s debugging toolkit. When applied proficiently, it offers authentic-time insights into how an software behaves, serving to you comprehend what’s taking place under the hood without needing to pause execution or stage with the code line by line.
A great logging technique starts with understanding what to log and at what stage. Widespread logging stages incorporate DEBUG, Data, WARN, ERROR, and Lethal. Use DEBUG for specific diagnostic facts during enhancement, Facts for normal functions (like profitable commence-ups), WARN for opportunity problems that don’t break the applying, ERROR for actual complications, and Lethal once the method can’t continue.
Stay clear of flooding your logs with excessive or irrelevant facts. Excessive logging can obscure crucial messages and slow down your process. Give attention to important situations, condition alterations, input/output values, and important determination points as part of your code.
Format your log messages Evidently and persistently. Consist of context, like timestamps, ask for IDs, and function names, so it’s much easier to trace concerns in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily useful in output environments in which stepping as a result of code isn’t doable.
In addition, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a effectively-believed-out logging technique, you can decrease the time it will require to identify problems, achieve further visibility into your applications, and improve the overall maintainability and reliability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it's a type of investigation. To properly determine and correct bugs, builders will have to method the procedure like a detective fixing a secret. This mindset allows stop working complicated concerns into manageable areas and observe clues logically to uncover the foundation cause.
Begin by gathering proof. Think about the symptoms of the issue: error messages, incorrect output, or efficiency troubles. The same as a detective surveys against the law scene, obtain just as much related details as it is possible to with no jumping to conclusions. Use logs, examination circumstances, and consumer reviews to piece with each other a clear picture of what’s taking place.
Up coming, kind hypotheses. Request oneself: What could possibly be leading to this conduct? Have any adjustments not too long ago been created to your codebase? Has this situation transpired prior to under similar instances? The target is usually to narrow down possibilities and recognize possible culprits.
Then, test your theories systematically. Try to recreate the situation in the controlled environment. For those who suspect a certain perform or ingredient, isolate it and validate if The problem persists. Like a detective conducting interviews, ask your code issues and Allow the results direct you closer to the reality.
Shell out close interest to small information. Bugs frequently disguise inside the least predicted places—just like a missing semicolon, an off-by-one particular error, or a race issue. Be thorough and client, resisting the urge to patch the issue devoid of totally knowledge it. Short-term fixes may perhaps conceal the real dilemma, just for it to resurface later.
And lastly, maintain notes on Anything you attempted and figured out. Equally as detectives log their investigations, documenting your debugging method can help you save time for long run issues and aid Many others comprehend your reasoning.
By imagining similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be simpler at uncovering concealed issues in sophisticated devices.
Write Exams
Composing assessments is among the simplest ways to enhance your debugging competencies and General advancement effectiveness. Assessments not simply assistance capture bugs early but also serve as a safety net that gives you self esteem when earning changes to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint precisely in which and when an issue takes place.
Get started with device assessments, which target specific features or modules. These modest, isolated exams can swiftly reveal whether or not a specific piece of logic is Operating as expected. When a exam fails, you straight away know wherever to glance, drastically minimizing time spent debugging. Device tests are Primarily handy for catching regression bugs—troubles that reappear right after previously being preset.
Following, integrate integration checks and conclusion-to-conclude tests into your workflow. These assist ensure that many portions of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex devices with many elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely Believe critically about your code. To test a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge scenarios. This degree of knowledge Normally potential customers to better code framework and much less bugs.
When debugging a concern, crafting a failing examination that reproduces the bug is usually a powerful initial step. As soon as the test fails continually, more info you can give attention to correcting the bug and watch your examination pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In brief, composing assessments turns debugging from the frustrating guessing recreation right into a structured and predictable course of action—aiding you capture additional bugs, faster and even more reliably.
Just take Breaks
When debugging a difficult difficulty, it’s easy to become immersed in the issue—watching your display screen for hrs, hoping Answer soon after Resolution. But Among the most underrated debugging applications is solely stepping absent. Having breaks allows you reset your intellect, reduce frustration, and often see the issue from the new standpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may begin overlooking apparent errors or misreading code that you simply wrote just hours before. During this point out, your Mind results in being a lot less successful at dilemma-fixing. A short wander, a espresso split, and even switching to a special task for ten–quarter-hour can refresh your target. Numerous developers report discovering the root of a dilemma once they've taken time for you to disconnect, letting their subconscious get the job done while in the track record.
Breaks also assist reduce burnout, In particular for the duration of for a longer time debugging classes. Sitting down before a screen, mentally trapped, is not merely unproductive but additionally draining. Stepping absent lets you return with renewed Power in addition to a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or maybe a misplaced variable that eluded you just before.
Should you’re trapped, a superb rule of thumb is usually to set a timer—debug actively for forty five–sixty minutes, then take a five–10 moment break. Use that time to maneuver close to, extend, or do one thing unrelated to code. It might sense counterintuitive, Specifically less than tight deadlines, but it surely really brings about quicker and simpler debugging in the long run.
In a nutshell, having breaks just isn't an indication of weakness—it’s a smart tactic. It provides your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight That always blocks your progress. Debugging is actually a psychological puzzle, and relaxation is part of fixing it.
Study From Each Bug
Each and every bug you encounter is much more than simply A short lived setback—it's an opportunity to increase for a developer. Whether or not it’s a syntax error, a logic flaw, or possibly a deep architectural challenge, every one can teach you some thing worthwhile when you take the time to mirror and examine what went Erroneous.
Get started by inquiring yourself a couple of crucial inquiries when the bug is solved: What brought about it? Why did it go unnoticed? Could it are actually caught before with improved practices like device tests, code assessments, or logging? The responses frequently reveal blind spots inside your workflow or comprehending and assist you to Develop more powerful coding routines shifting forward.
Documenting bugs can also be a wonderful pattern. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you realized. With time, you’ll start to see patterns—recurring challenges or prevalent problems—which you could proactively stay away from.
In group environments, sharing what you've acquired from the bug along with your peers is usually In particular effective. Regardless of whether it’s through a Slack information, a brief compose-up, or A fast know-how-sharing session, aiding Other people steer clear of the very same problem boosts workforce effectiveness and cultivates a stronger Mastering tradition.
More importantly, viewing bugs as classes shifts your state of mind from annoyance to curiosity. As an alternative to dreading bugs, you’ll start appreciating them as necessary portions of your advancement journey. After all, several of the very best builders aren't those who write best code, but those that repeatedly discover from their faults.
In the end, Every single bug you fix adds a completely new layer in your talent established. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional capable developer as a result of it.
Summary
Improving your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you happen to be knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become far better at That which you do.